Java में Operators का उपयोग variables और values पर operations करने के लिए किया जाता है। Operators को 6 प्रकारों में विभाजित किया गया है:: Arithmetic, Comparison, Logical, Assignment Operator
Types of Java Operators
Java Operators को 6 प्रकारों में विभाजित किया गया है:
- Arithmetic operators
- Comparison operators
- Logical operators
- Assignment operators
- Bitwise operators
- Misc Operators
Arithmetic Operators
Arithmetic Java Operators का इस्तेमाल common और simple mathematical calculation को करने के लिए किया जाता हैं
Operator | Name | Description | Example |
---|---|---|---|
+ | Addition | Adds together two values | x + y |
- | Subtraction | Subtracts one value from another | x - y |
* | Multiplication | Multiplies two values | x * y |
/ | Division | Divides one value by another | x / y |
% | Modulus | Returns the division remainder | x % y |
++ | Increment | Increases the value of a variable by 1 | ++x |
-- | Decrement | Decreases the value of a variable by 1 | --x |
public class MyClass {
public static void main(String[] args) {
int num1 = 10;
int num2 = 5;
int num3 = num1 + num2;
System.out.println(num1);
System.out.println(3 + num1);
System.out.println(num3);
}
}
// Result for num1 : 10
// Result for 3 + num1 : 13
// Result for num3 : 15
public class MyClass {
public static void main(String[] args) {
int x = 20;
int y = 5;
System.out.println(x + y); // Result: 25
System.out.println(x - y); // Result: 15
System.out.println(x * y); // Result: 100
System.out.println(x / y); // Result: 4
System.out.println(x % 3); // Result: 2
}
}
public class MyClass {
public static void main(String[] args) {
int x = 20;
int y = 5;
++x;
--y;
System.out.println(x); // Result: 21
System.out.println(y); // Result: 4
}
}
उपरोक्त उदाहरण में, Increment operator ++ या -- का उपयोग, variable के प्रति prefix या suffix के रूप में किया जा सकता है।
Comparison Operators
Comparison Java Operators: जैसे के नाम से ही समझ सकते है, यह दो वैल्यू की तुलना करता है और परिणाम true या false में देता है।
Operator | Name | Example |
---|---|---|
== | Equal to | x == y |
!= | Not equal | x != y |
> | Greater than | x > y |
< | Less than | x < y |
>= | Greater than or equal to | x >= y |
<= | Less than or equal to | x <= y |
public class MyClass {
public static void main(String[] args) {
int x = 8;
int y = 5;
System.out.println(x == y); // Result: false
System.out.println(x != y); // Result: true
System.out.println(x > y); // Result: true
System.out.println(x < y); // Result: false
System.out.println(x >= y); // Result: true
System.out.println(x <= y); // Result: false
}
}
Logical Operators
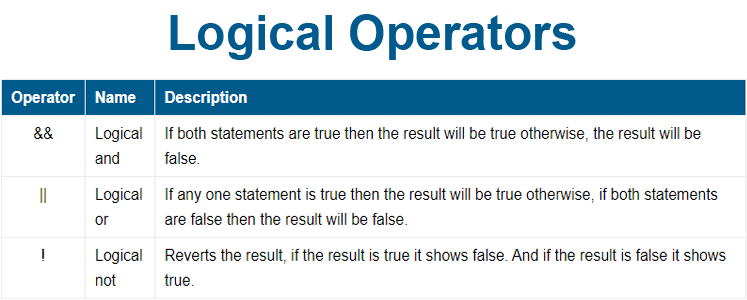
Logical operators का इस्तेमाल दो variables के बिच के वैल्यू के logic जानने के लिए किया जाता हैं।
Operator | Name | Description |
---|---|---|
&& | Logical and | यदि दोनों statements सत्य हैं, तो true दिखाता है, अन्यथा false दिखाता हैं। |
|| | Logical or | यदि एक भी statements सत्य हैं, तो true दिखाता है, अन्यथा अगर दोनों statements असत्य हैं तो false दिखाता हैं । |
! | Logical not | Result को उल्टा कर देता हैं, यदि परिणाम true है तो यह उसे false दिखाता है, यदि परिणाम false है तो यह उसे true दिखाता है। |
public class MyClass {
public static void main(String[] args) {
int x = 8;
System.out.println(x > 5 && x == 8);
// Result: true
System.out.println(x > 5 || x != 8);
// Result: true
System.out.println(!(x > 5 && x == 8));
// Result: fale
}
}
Assignment Operators
Assignment operators इस्तेमाल होता है variables को कूछ values नियूक्त करने के लिए।
सभी Assignment operators की एक सूची नीचे दी गयी हैं।
Operator | Example | Same As |
---|---|---|
= | x += 5 | x = x + 5 |
+= | x += 5 | x = x + 5 |
-= | x -= 5 | x = x - 5 |
*= | x *= 5 | x = x * 5 |
/= | x /= 5 | x = x / 5 |
%= | x %= 5 | x = x % 5 |
&= | x &= 5 | x = x & 5 |
|= | x |= 3 | x = x | 3 |
^= | x ^= 3 | x = x ^ 3 |
>>= | x >>= 3 | x = x >> 3 |
<<= | x <<= 3 | x = x << 3 |
public class MyClass {
public static void main(String[] args) {
int x = 5;
x += 3;
System.out.println(x); // Result: 8
}
}
public class MyClass {
public static void main(String[] args) {
int x = 5;
x = x + 3;
System.out.println(x); // Result: 8
}
}
ऊपर दिए गए उदाहरण से आप समझ गए होंगे Assignment operators दोनों तरीको से काम कर सकता है, और उनका result एक जैसा ही होता हैं।
Bitwise Operators
Java में कई bitwise operators है, जिन्हें integer data types जैसे की byte, short, int और long पर इस्तेमाल किया जाता हैं। इस तरह के operator bits पर काम करते है और bit-by-bit कर के operation को अंजाम देते हैं।
मान लेते हैं की एक integer डाटा टाइप का variable x = 60 और y = 13 हैं।
Operator | Description | Example |
---|---|---|
& | Binary AND Operator copies a bit to the result if it exists in both operands. | (x & y) will give 12 which is 0000 1100 |
| | Binary OR Operator copies a bit if it exists in either operand. | (x | y) will give 61 which is 0011 1101 |
^ | Binary XOR Operator copies the bit if it is set in one operand but not both. | (x ^ y) will give 49 which is 0011 0001 |
~ | Binary One's Complement Operator is unary and has the effect of 'flipping' bits. | (~x ) will give -61 which is 1100 0011 in 2's complement form due to a signed binary number. |
<< | Binary Left Shift Operator. The left operands value is moved left by the number of bits specified by the right operand. | x << 2 will give 240 which is 1111 0000 |
>> | Binary Right Shift Operator. The left operands value is moved right by the number of bits specified by the right operand. | x >> 2 will give 15 which is 1111 |
>>> | Shift right zero-fill operator. The left operands value is moved right by the number of bits specified by the right operand and shifted values are filled up with zeros. | x >>>2 will give 15 which is 0000 1111 |