PHP Complete Form चैप्टर में real-time फॉर्म, actions के साथ देखेंगे। नीचे दिया गया उदाहरण इनपुट फ़ील्ड को text, radio button और checked box के रूप में लेने के साथ-साथ डेटा को validate भी करेगा।
PHP Complete Form
<!DOCTYPE html>
<html>
<head>
<style>
.error {
color: #FF0000;
}
</style>
</head>
<body>
<?php
// कई variables बनाया और उसको empty values सेट कर दिया
$nameErr = $emailErr = $websiteErr = $mobilenoErr = $genderErr = $agreeErr = "";
$name = $email = $website = $mobileno = $gender = $comment = $agree = "";
//Input fields validation
if ($_SERVER["REQUEST_METHOD"] == "POST") {
//Name Validation
if (empty($_POST["name"])) {
$nameErr = "Name is required";
} else {
$name = input_data($_POST["name"]);
// check if name only contains letters and whitespace
if (!preg_match("/^[a-zA-Z-' ]*$/", $name)) {
$nameErr = "Only letters and white space allowed";
}
}
//E-mail ID Validation
if (empty($_POST["email"])) {
$emailErr = "Email is required";
} else {
$email = input_data($_POST["email"]);
// Check if e-mail address is well-formed
if (!filter_var($email, FILTER_VALIDATE_EMAIL)) {
$emailErr = "Invalid email format";
}
}
//Website URL Validation
if (empty($_POST["website"])) {
$website = "";
} else {
$website = input_data($_POST["website"]);
// check if URL address syntax is valid
if (!preg_match("/\b(?:(?:https?|ftp):\/\/|www\.)[-a-z0-9+&@#\/%?=~_|!:,.;]*[-a-z0-9+&@#\/%=~_|]/i", $website)) {
$websiteErr = "Invalid URL";
}
}
//Mobile Number Validation
if (empty($_POST["mobileno"])) {
$mobilenoErr = "Mobile no is required";
} else {
$mobileno = input_data($_POST["mobileno"]);
// check if mobile no is well-formed
if (!preg_match("/^[0-9]*$/", $mobileno)) {
$mobilenoErr = "Only numeric value is allowed";
}
//check mobile no length should be 10 digit
if (strlen($mobileno) != 10) {
$mobilenoErr = "Mobile no must contain 10 digits";
}
}
//Radio Button Gender
if (empty($_POST["gender"])) {
$genderErr = "Gender is required";
} else {
$gender = input_data($_POST["gender"]);
}
//Comment Form
if (empty($_POST["comment"])) {
$comment = "";
} else {
$comment = input_data($_POST["comment"]);
}
//Checkbox Validation
if (!isset($_POST["agree"])) {
$agreeErr = "Accept terms of services before submit";
} else {
$agree = input_data($_POST["agree"]);
}
}
function input_data($data)
{
$data = trim($data);
$data = stripslashes($data);
$data = htmlspecialchars($data);
return $data;
}
?>
<h2>PHP Complete Form</h2>
<p><span class="error">* Required Field</span></p>
<form method="post" action="">
Name:
<input type="text" name="name" value="<?php echo $name; ?>">
<span class="error">*<?php echo $nameErr; ?></span>
<br><br>
E-mail:
<input type="text" name="email" value="<?php echo $email; ?>">
<span class="error">*<?php echo $emailErr; ?></span>
<br><br>
Website:
<input type="text" name="website" value="<?php echo $website; ?>">
<span class="error"><?php echo $websiteErr; ?></span>
<br><br>
Mobile No:
<input type="text" name="mobileno" value="<?php echo $mobileno; ?>">
<span class="error">*<?php echo $mobilenoErr; ?></span>
<br><br>
Gender:
<input type="radio" name="gender" <?php if (isset($gender) && $gender == "male") echo "checked"; ?> value="Male">Male
<input type="radio" name="gender" <?php if (isset($gender) && $gender == "female") echo "checked"; ?> value="Female">Female
<input type="radio" name="gender" <?php if (isset($gender) && $gender == "other") echo "checked"; ?> value="Other">Other
<span class="error">* <?php echo $genderErr; ?></span>
<br><br>
Comment:
<textarea name="comment" rows="5" cols="40"><?php echo $comment; ?></textarea>
<br><br>
Agree to Terms of Service: <input type="checkbox" name="agree">
<span class="error">* <?php echo $agreeErr; ?></span>
<br> <br>
<input type="submit" name="submit" value="Submit">
</form>
<?php
echo "<h2>Output Result:</h2>";
echo "Name: " . $name . "<br>";
echo "E-mail: " . $email . "<br>";
echo "Website: " . $website . "<br>";
echo "Mobile No: " . $mobileno . "<br>";
echo "Gender: " . $gender . "<br>";
echo "Comment: " . $comment;
?>
</body>
</html>
उपरोक्त कोड अधिकांश यूजर द्वारा उपयोग किए जाने वाले फॉर्म का नमूना है।
नीचे दी गई image, उपरोक्त कोड का UI है।
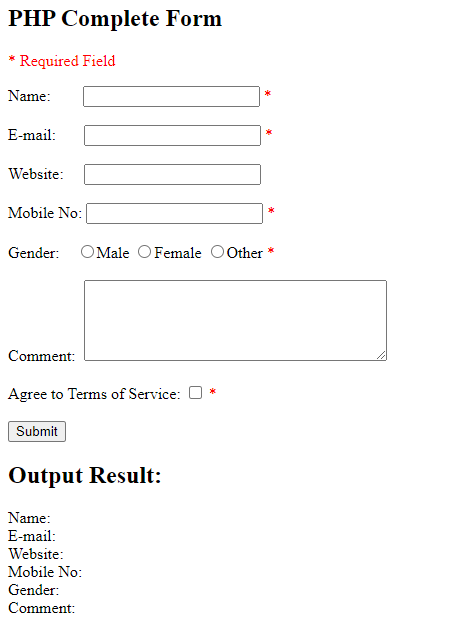