Java Condition if .. else statement, का उपयोग दी गई कंडिशन true है या false है चेक करने लिए किया जाता है और उन्ही के अनुसार code को execute कराने के लिए किया जाता है।
Java mathematics के, basic logical conditions को ही सपोर्ट करता है। जैसा की आप निचे देख सकते है।
- Less than: x < y
- Greater than: x > y
- Less than or equal to: x <= y
- Greater than or equal to: x >= y
- Equal to: x == y
- Not Equal to: x != y
Java के पास निम्नलिखित conditional statements हैं।
- if statement
- else statement
- else if statement
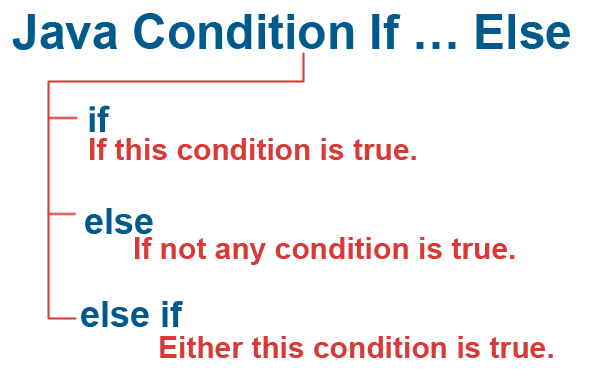
Java Condition if Statement
if
statement का उपयोग, यदि condition true है तो code block को execute करने के लिए किया जाता है। और यदि condition झूठी अर्थात false है तो इसे execute नहीं किया जाएगा।
public class MyClass {
public static void main(String[] args) {
int x = 9;
if (x < 15) {
System.out.println("Correct Answer");
}
}
}
// Result: Correct Answer
उपरोक्त उदाहरण में, यह result दिखाता है क्योंकि condition सत्य है क्योंकि x यानी 9 का वैल्यू 15 से कम है।
public class MyClass {
public static void main(String[] args) {
int x = 9;
if (x > 15) {
System.out.println("No result disply");
}
}
}
/* In this nothing will display
as the condition is false. */
उपरोक्त उदाहरण में, condition false है इसका मतलब है की यह कोई रिजल्ट नहीं दिखाएगा।
else Statement
उपरोक्त उदाहरणों से हमें पता चलता है कि यदि Java condition, true है तो यह execute हो जाता है और कुछ ऐसा प्रिंट करता है जो हम चाहते हैं। लेकिन अगर condition, false है तो भी हमें कुछ और प्रिंट करने की जरूरत है। उसके लिए, हम else
statements का उपयोग करते हैं।
public class MyClass {
public static void main(String[] args) {
int x = 9;
if (x > 15) {
System.out.println("Correct Answer");
} else {
System.out.println("Wrong Condition");
}
}
}
// Result: Wrong Condition
उपरोक्त उदाहरण में, Condition false है क्योंकि x का वैल्यू यानी की 9, 15 से अधिक नहीं है, इसलिए रिजल्ट में else
statement के code block प्रिंट होता है।
इसको इस तरह से समझते हैं।
1st Check: if
statement की जाँच होती है, अगर कंडीशन true होगी तो code block को execute करेगी, और execute करते ही वही से बाहर निकल जाएगी। और if
statement से रिजल्ट दे देगी।
2nd Check: सबसे पहले if
statement की जाँच होती है, अगर कंडीशन false होगी तो, java अगले statement यानि की else
statement में चली जाएगी और की जाँच करेगी और else
statement से रिजल्ट प्रिंट करा देगी।
else if Statement
यदि पहली condition false है तो यह अगली condition की जाँच करता है, इसके लिए else if
statement का उपयोग किया जाता है। आइए नीचे से एक उदाहरण से समझते हैं।
public class MyClass {
public static void main(String[] args) {
int x = 9;
if (x > 15) {
System.out.println("Ans - x is greater");
} else if (x == 9) {
System.out.println("Ans - x is equal to 9");
} else {
System.out.println("Wrong Condition");
}
}
}
// Result: Ans - x is equal to 9
आसान भाषा में समझते हैं
if= अगर यह सही है तो (Condition)
else if= या तो यह सही है (Condition)
else= जब ऊपर का कोई भी सही नहीं है।